Previously, I showed how to solve a simple problem of motion at a constant velocity analytically and numerically. Because of the nature of the problem both solutions gave the same result. Now we’ll try a constant acceleration problem which should highlight some of the key differences between the two approaches, particularly the tradeoffs you must make when using numerical approaches.
The Problem
- A ball starts at the origin and moves horizontally with an acceleration of 0.2 m/s2. Print out a table of the ball’s position (in x) with time (every second) for the first 20 seconds.
Analytical Solution
We know that acceleration (a) is the change in velocity with time (t):
so if we integrate acceleration we can find the velocity. Then, as we saw before, velocity (v) is the change in position with time:
which can be integrated to find the position (x) as a function of time.
So, to summarize, to find position as a function of time given only an acceleration, we need to integrate twice: first to get velocity then to get x.
For this problem where the acceleration is a constant 0.2 m/s2 we start with acceleration:
which integrates to give the general solution,
To find the constant of integration we refer to the original question which does not say anything about velocity, so we assume that the initial velocity was 0: i.e.:
at t = 0 we have v = 0;
which we can substitute into the velocity equation to find that, for this problem, c is zero:
making the specific velocity equation:
we replace v with dx/dt and integrate:
This constant of integration can be found since we know that the ball starts at the origin so
at t = 0 we have x = 0, so;
Therefore our final equation for x is:
Summarizing the Analytical
To summarize the analytical solution:
These are all a function of time so it might be more proper to write them as:
Velocity and acceleration represent rates of change which so we could also write these equations as:
or we could even write acceleration as the second differential of the position:
or, if we preferred, we could even write it in prime notation for the differentials:
The Numerical Solution
As we saw before we can determine the position of a moving object if we know its old position (xold) and how much that position has changed (dx).
where the change in position is determined from the fact that velocity (v) is the change in position with time (dx/dt):
which rearranges to:
So to find the new position of an object across a timestep we need two equations:
In this problem we don’t yet have the velocity because it changes with time, but we could use the exact same logic to find velocity since acceleration (a) is the change in velocity with time (dv/dt):
which rearranges to:
and knowing the change in velocity (dv) we can find the velocity using:
Therefore, we have four equations to find the position of an accelerating object (note that in the third equation I’ve replaced v with vnew which is calculated in the second equation):
These we can plug into a python program just so:
motion-01-both.py
from visual import * # Initialize x = 0.0 v = 0.0 a = 0.2 dt = 1.0 # Time loop for t in arange(dt, 20+dt, dt): # Analytical solution x_a = 0.1 * t**2 # Numerical solution dv = a * dt v = v + dv dx = v * dt x = x + dx # Output print t, x_a, x
which give output of:
>>> 1.0 0.1 0.2 2.0 0.4 0.6 3.0 0.9 1.2 4.0 1.6 2.0 5.0 2.5 3.0 6.0 3.6 4.2 7.0 4.9 5.6 8.0 6.4 7.2 9.0 8.1 9.0 10.0 10.0 11.0 11.0 12.1 13.2 12.0 14.4 15.6 13.0 16.9 18.2 14.0 19.6 21.0 15.0 22.5 24.0 16.0 25.6 27.2 17.0 28.9 30.6 18.0 32.4 34.2 19.0 36.1 38.0 20.0 40.0 42.0
Here, unlike the case with constant velocity, the two methods give slightly different results. The analytical solution is the correct one, so we’ll use it for reference. The numerical solution is off because it does not fully account for the continuous nature of the acceleration: we update the velocity ever timestep (every 1 second), so the velocity changes in chunks.
To get a better result we can reduce the timestep. Using dt = 0.1 gives final results of:
18.8 35.344 35.532 18.9 35.721 35.91 19.0 36.1 36.29 19.1 36.481 36.672 19.2 36.864 37.056 19.3 37.249 37.442 19.4 37.636 37.83 19.5 38.025 38.22 19.6 38.416 38.612 19.7 38.809 39.006 19.8 39.204 39.402 19.9 39.601 39.8 20.0 40.0 40.2
which is much closer, but requires a bit more runtime on the computer. And this is the key tradeoff with numerical solutions: greater accuracy requires smaller timesteps which results in longer runtimes on the computer.
Post Script
To generate a graph of the data use the code:
from visual import * from visual.graph import * # Initialize x = 0.0 v = 0.0 a = 0.2 dt = 1.0 analyticCurve = gcurve(color=color.red) numericCurve = gcurve(color=color.yellow) # Time loop for t in arange(dt, 20+dt, dt): # Analytical solution x_a = 0.1 * t**2 # Numerical solution dv = a * dt v = v + dv dx = v * dt x = x + dx # Output print t, x_a, x analyticCurve.plot(pos=(t, x_a)) numericCurve.plot(pos=(t,x))
which gives:
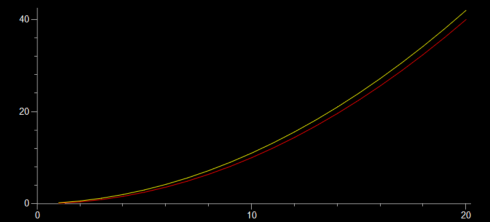